Office Proximity Sensor
I love my QC35s at work. Except when someone sneaks up behind me. This is a proximity sensor project to detect when someone is walking up behind me. The idea is to turn on a led that's in my field of view for a few seconds when someone walks up.
I started first with a PIR sensor that I had around from a previous project. Much like this
one.
Didn't work very well at first, was flaky and erratic. Turns out, it needs 12V which I didn't have as part of the RPI. ...And I was also too lazy to go get a 12V supply.
Turns out though that I did have a ultra-sonic range finder. I also found this
tutorial for hooking it up.
Here's the initial prototype of using the range finder to at least detect range.
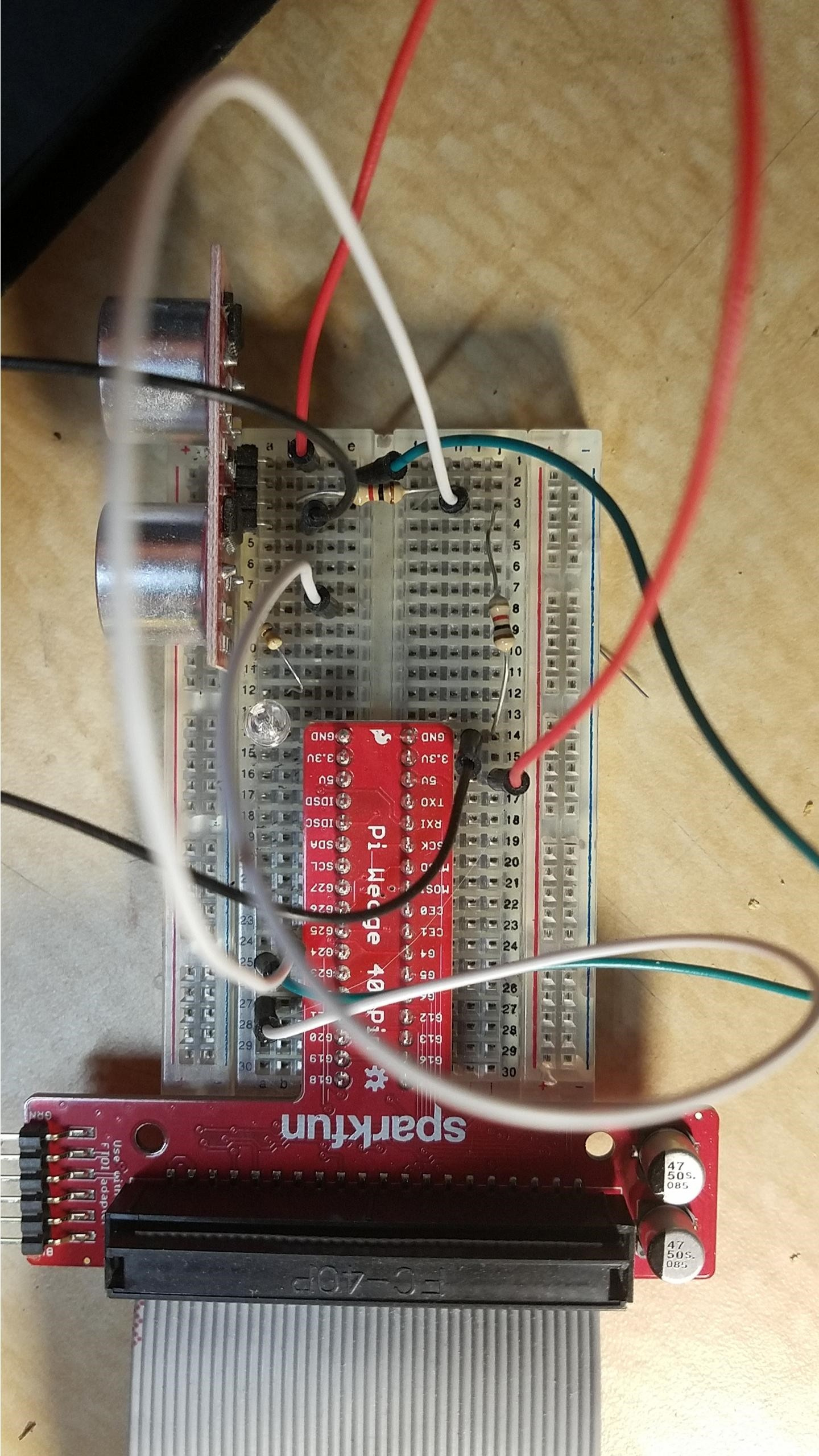
A few things I wanted to add around the basic structure provided in the tutorial. Once you learn how to use the RPI GPIO they are pretty easy.
- LED for indication
- A method for changing the detection range
LED
A few years ago for Halloween, I bought these LEDs of Amazon. They are pretty cool. All you need to do is bias them an the LED blinks RGB. They will definitely get your attention.
Range Selection
I had an idea of the width of the opening of my cube, but I also figured that once I mounted the sensor, the actual range that a person would be within could change. I went back to my random part bins and found a 6position dip switch. Instead of doing a binary range based on the value of the dip (which is something I might still go back and do) I just used it more like a decade box I add 40cm of range per bit position set. Position 1 = 40cm, 1 and 2 = 80 cm, and so on. Not super complicated, and if you look at the code, it just picks up on the first position it finds so technically if you only set position 2, then you only get 40cm. I agree, lazy, but it's a hobby, not a product.
If you look at the datasheet, the range of the sensor is really around 2m, so that's my max value.
Running on the RPi
On the RPi, this is all running as a python script. for testing, its as simple as navigating to where you have the files tored and running
> python directory/scriptname.py
To get this to run automatically (especially for a headless RPi) I modified /etc/rc.local to include the line above command line.
Proto Card Build
Once the circuit was proven on the breadboard, I moved it to a RPI prototype board for a cleaner fit. Yes, the lid cut outs are kinda hack, but I don't have a CNC yet and it was too late to use the Dremmel without waking the house.
Schematic
CODE LISTING
#sensor.py
import RPi.GPIO as GPIO
import time
import sys
GPIO.setmode(GPIO.BCM)
#GPIO.cleanup()
TRIG=23
ECHO=24
#range select inputs
RANGE0=4
RANGE1=17
RANGE2=18
RANGE3=27
RANGE4=22
#configure gpio direction
GPIO.setup(TRIG,GPIO.OUT)
GPIO.setup(ECHO,GPIO.IN)
GPIO.setup(RANGE0,GPIO.IN,pull_up_down=GPIO.PUD_UP)
GPIO.setup(RANGE1,GPIO.IN,pull_up_down=GPIO.PUD_UP)
GPIO.setup(RANGE2,GPIO.IN,pull_up_down=GPIO.PUD_UP)
GPIO.setup(RANGE3,GPIO.IN,pull_up_down=GPIO.PUD_UP)
GPIO.setup(RANGE4,GPIO.IN,pull_up_down=GPIO.PUD_UP)
GPIO.setup(21,GPIO.OUT) #LED
distance=1000 #set high
pulse_start=0
pulse_end=0
detection_Range = 0.00 #distance in centementers
try:
print("DISTANCE MEASUREMENT IN PROGRESS")
GPIO.output(TRIG,False)
GPIO.output(21,False)
print ("waiting for sensor to settle")
time.sleep(2)
while True:
print ("gpio status: ", GPIO.input(RANGE4), GPIO.input(RANGE3),GPIO.input(RANGE2), GPIO.input(RANGE1), GPIO.input(RANGE0))
detection_Range = 0
if GPIO.input(RANGE0)==1:
detection_Range+=40
if GPIO.input(RANGE1)==1:
detection_Range+=40
if GPIO.input(RANGE2)==1:
detection_Range+=40
if GPIO.input(RANGE3)==1:
detection_Range+=40
if GPIO.input(RANGE4)==1:
detection_Range+=40
time.sleep(.1)
GPIO.output(TRIG,True)
time.sleep(0.00001)
GPIO.output(TRIG,False)
while GPIO.input(ECHO)==0:
pulse_start=time.time()
while GPIO.input(ECHO)==1:
pulse_end=time.time()
pulse_duration=pulse_end-pulse_start
distance=pulse_duration*17150
distance=round(distance,2)
print ("Distance:", distance, "cm")
print ("Distance Range:", detection_Range)
if (distance GPIO.output(21,True)
time.sleep(3)
else:
GPIO.output(21,False)
time.sleep(1)
#zero everything out
pulse_start=0
pulse_end=0
distance=1000
GPIO.output(21,False)
except:
GPIO.cleanup()
Copyright 2020 DVLANG